Get started
API Endpoint https://api.starspaceshop.com/rapidpay_api/
Welcome to the Rapidpays API! Our API can let you access to Rapidpays API endpoint to sending bill to your customer in order to make payment.
Our API is organized around REST. JSON will be returned in all responses from the API, including errors. The API will be accepted form-data with POST method.
To use this API, you need an API key. Please contact us at [email protected] to get your own API key.
create a bill
# Here is a curl example
curl \
-X POST http://api.starspaceshop.com/rapidpay_api/create_bill/ \
-F 'secret_key=your_api_key' \
-F 'customer_code=P0001' \
-F 'amount=100' \
-F 'return_url=your_return_url' \
-F 'callback_url=your_callback_url' \
-F 'bank_key=your_bank_key' \
-F 'remark=Rapidpays testing API' \
Note: If you are using rapidpays bank, then bank_key no need to pass. Else bank_key is required.
Result example :
result: [
{
"customer_code": "P0001",
"transaction_id": "TT_1",
"transaction_date": "2021-09-29 14:46:23",
"payment_link": "https://bill.rapidpays.com?id=TT_1&s=43ef77ba0ad2bd2de8ee2eb76aa6dd472a398616",
"status": "SUCCESS",
"message": "bill created successful."
}
]
}
QUERY PARAMETERS
Field | Type | Description |
---|---|---|
secret_key | String | Your API key. |
customer_code | String | Your customer code. This customer code have to generate by merchant side and pass to us. It's use to recognize which transaction from which customer. |
amount | Double(10,2) | Your transaction amount. |
return_url | String | Return url is bring the customer to which merchant page when customer done make transaction. |
callback_url | String | Callback url is to let merchant know the status of transaction. |
customer_name | String | (Optional) Your customer name. Pass this param will enable name verification for Online Banking service. |
holder_name | String | (Optional) Your customer name. Pass this param will direct show entered holder name in payment page for QR service. |
bank_key | String | (Optional) Your bank key, if you have more than one bank, you can choose which bank you want the transaction transfer to. |
remark | String | (Optional) You can put your remark here. |
bank_code | String | (Optional) You can direct put the bank code here to bring the customer direct to specific bank page. Please refer to Payment Bank |
RESPONSE PARAMETERS
Field | Type | Description |
---|---|---|
customer_code | String | This customer code is generated by merchant and merchant have to save down the code for customer next time transaction. |
transaction_id | String | This is your transaction ID to verify or check the transaction status. |
transaction_date | Datetime | Your transaction amount. |
payment_link | String | This is payment link that you have to redirect customer go to. |
status | String | This is the status for the API call, mostly will have SUCCESS or ERROR |
message | String | Here will print out the error or success message when you call the API. |
check a bill
# Here is a curl example
curl \
-X POST http://api.starspaceshop.com/rapidpay_api/check_bill \
-F 'transaction_id=TT_1' \
Result example :
result: [
{
"status": "SUCCESS",
"transaction_id": "TT_1",
"customer_code": "P0001",
"amount": "100.00",
"transaction_date": "2021-09-29 14:46:23",
"bank_to": "afe4c43a096e23f69ade664002c364595fc5b5ba",
"bank_time": null,
"reference_number": null,
"remark": "testing purpose only",
"return_url": "https://www.rapidpays.com",
"callback_url": "https://www.rapidpays.com",
"state": "PENDING"
}
]
}
QUERY PARAMETERS
Field | Type | Description |
---|---|---|
transaction_id | String | Your transaction id. |
RESPONSE PARAMETERS
Field | Type | Description |
---|---|---|
bank_time | Datetime | The transaction complete datetime |
reference_number | String | Bank to bank reference number |
state | ENUM(PENDING, SUCCESS, FAILED) | This is the status for transaction. |
Transaction Callback Response
Here is the sample callback response from system upon transaction completion (success/fail)
Response example :
{
"ID": "TT_1402228",
"CustomerCode": "ZYT1023523",
"Amount": 20,
"Status": "success",
"Message": "Transaction Successful",
"ReferenceNumber": "3941915294",
"BankTime": "2023-04-10 14:49:00",
"Hash": "abcd1234"
}
RESPONSE PARAMETERS
Field | Type | Description |
---|---|---|
ID | String | Your transaction id |
Status | ENUM(success, fail) | Status for transaction |
Hash | String | This is the signature of verifying transaction. It's using sha1 encryption. Here is the format of transaction verification: sha1(ID + CustomerCode + Amount + Status + SecretKey) |
add bank
# Here is a curl example
curl \
-X POST http://api.starspaceshop.com/rapidpay_api/add_bank/ \
-F 'secret_key=your_api_key' \
-F 'bank_holder_name=testing' \
-F 'bank_account=1234567888' \
-F 'bank_code=MayBank' \
-F 'tran_limit=0' \
-F 'tran_count=0' \
Every add bank action will be temporary in PENDING status, it have to wait our staff to verify and approve.
Result example :
result: [
{
"bank_code": "MayBank",
"bank_holder_name": "testing",
"bank_account": "1234567888",
"type": "NORMAL",
"tran_limit": "0",
"tran_count": "0",
"isActive": "Y",
"state": "PENDING",
"status": "SUCCESS",
"message": "Bank has been added successful, please wait for verification.",
"bank_key": "44e79aa1ec87be10c8546d1388a716d52b114379"
}
]
}
QUERY PARAMETERS
Field | Type | Description |
---|---|---|
secret_key | String | Your API key. |
bank_holder_name | String | Your bank holder name |
bank_account | String | Your bank account |
bank_code | String | Please refer to Bank Code Table. |
tran_limit (OPTIONAL) | Double(10,2) | Transaction Limit is to control your bank daily transaction, by default is 0 (unlimited), you can self change the limit like daily receive total transaction amount with 1,000 then Rapidpays will check either Transaction Limit or Transaction Count reach the limit first. |
tran_count (OPTIONAL) | Integer | Transaction Count is to check the count of daily transaction, by default is 0 (unlimited), you can change the count with 100 which mean Rapidpays will check either Transaction Limit or Transaction Count reach the limit. |
RESPONSE PARAMETERS
Field | Type | Description |
---|---|---|
bank_code | String | Please refer to Bank Code Table |
bank_holder_name | String | Your bank holder name |
bank_account | String | Your bank account |
state | String | This is your bank status, have to wait until our verification finish. |
bank_key | String | Your bank key |
update bank
# Here is a curl example
curl \
-X POST http://api.starspaceshop.com/rapidpay_api/update_bank/ \
-F 'secret_key=your_api_key' \
-F 'bank_key=your_bank_key' \
-F 'isActive=ACTIVE' \
-F 'tran_limit=10000' \
-F 'tran_count=100' \
Result example :
result: [
{
"status": "SUCCESS",
"message": "Bank update successfully"
}
]
}
QUERY PARAMETERS
Field | Type | Description |
---|---|---|
secret_key | String | Your API key. |
bank_key | String | Your bank key |
isActive | ENUM(ACTIVE, INACTIVE) | (OPTIONAL) If you want to temporary stop the bank to receive payment, you can update your bank to INACTIVE. |
tran_limit | double(10,2) | (OPTIONAL) Update your bank transaction limit |
tran_count | integer | (OPTIONAL) Update your transaction count. |
Request Withdrawal
# Here is a curl example
curl \
-X POST http://api.starspaceshop.com/rapidpay_api/create_payout/ \
-F 'secret_key=your_api_key' \
-F 'unique_id=your_system_withdrawal_order_number' \
-F 'amount=100' \
-F 'callback_url=your_callback_url' \
-F 'customer_bank_code=MayBank' \
-F 'customer_bank_holder_name=TEST' \
-F 'customer_bank_acc=123456789012' \
To request withdrawal you need to make a POST call to the following url :
http://api.starspaceshop.com/rapidpay_api/create_payout/
Result example :
result: [
{
"payout_id": "TT_3",
"payout_date": "2022-04-14 17:09:33",
"amount": "10.00",
"totalamount": "10.00",
"status": "SUCCESS",
"message": "Payout created successful."
}
]
}
QUERY PARAMETERS
Field | Type | Description |
---|---|---|
secret_key | String | Your API key. |
unique_id | String | Your system withdrawal order number. |
amount | Double(10,2) | Your withdrawal amount. |
callback_url | String | Callback url is to let merchant know the status of withdrawal. |
customer_bank_code | String | Please refer to Withdraw Bank Table |
customer_bank_holder_name | String | Customer bank holder name |
customer_bank_acc | String | Customer bank account |
check withdrawal
# Here is a curl example
curl \
-X POST http://api.starspaceshop.com/rapidpay_api/check_payout \
-F 'payout_id=TT_1' \
To check a bill you need to make a POST call to the following url :
http://api.starspaceshop.com/rapidpay_api/check_payout/
Result example :
result: [
{
"status": "SUCCESS",
"payout_id": "TT_1",
"amount": "100.00",
"withdrawal_date": "2021-09-29 14:46:23",
"bank_time": null,
"remark": "testing purpose only",
"callback_url": "https://www.rapidpays.com",
"state": "PENDING"
}
]
}
QUERY PARAMETERS
Field | Type | Description |
---|---|---|
payout_id | String | Your payout id. |
RESPONSE PARAMETERS
Field | Type | Description |
---|---|---|
bank_time | Datetime | The withdrawal complete datetime |
state | ENUM(PENDING, SUCCESS, FAILED) | This is the status for withdrawal. |
Withdrawal Callback Response
Here is the sample callback response from system upon withdrawal completion (success/fail)
Response example :
{
"ID": "TT_1402228",
"UniqueID": TT_1,
"Amount": 20,
"Status": "success",
"Message": "Payout Successful",
"Hash": "abcd1234"
}
RESPONSE PARAMETERS
Field | Type | Description |
---|---|---|
ID | String | Your withdrawal id |
Status | ENUM(success, fail) | Status for withdrawal |
Hash | String | This is the signature of verifying withdrawal. It's using sha1 encryption. Here is the format of withdrawal verification: sha1(ID + Amount + Status + SecretKey) |
Bank Code Table
Every bank has a unique bank code. It is used to add your bank for deposit.
Malaysia
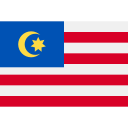
BANK TRANSFER
Bank | Code |
---|---|
PUBLIC BANK | PbeBank |
MAYBANK | MayBank |
CIMB BANK | CimbBank |
RHB BANK | RhbBank |
BANK RAKYAT | BankRakyat |
BANK ISLAM | BankIslam |
AM BANK | AmBank |
HONG LEONG BANK | HlbBank |
BANK SIMPANAN NASIONAL (BSN) | BSN |
ALLIANCE BANK | AllianceBank |
AFFIN BANK | AffinBank |
HSBC BANK | HSBCBank |
AGRO BANK | AgroBank |
STANDARD CHARTERED BANK | SCBank |
MUAMALAT BANK | MuamalatBank |
UOB BANK | UOBBank |
OCBC BANK | OCBCBank |
AL-RAJHI BANK | ARBank |
CITI BANK | CitiBank |
CDM | CDM |
MBSB BANK | MBSBBank |
QRPAY
Bank | Code |
---|---|
TOUCH'N GO | Tng |
MAYBANK QRPAY | MayBankQR |
DUITNOW QR / E-WALLET PAYMENT | DuitNowQR |
RHB BANK QRPAY | RhbBankQR |
GXBANK | GXBank |
GRABPAY | GrabPay |
BOOST | Boost |
TELCO
Bank | Code |
---|---|
DIGI | Digi |
UMOBILE | Umobile |
TELCOPIN | TelcoPIN |
MAXIS | Maxis |
CELCOM | Celcom |
TNG RELOAD PIN | TngRP |
Thailand
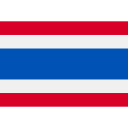
BANK TRANSFER
Bank | Code |
---|---|
KASIKORN BANK (KBANK) | KBank |
KRUNG THAI BANK (KTB) | KrungThaiBank |
SIAM COMMERCIAL BANK | SCBBank |
KRUNGSRI BANK (BAY) | KrungsriBank |
BANGKOK BANK | BangkokBank |
BANK FOR AGRICULTURE AND AGRICULTURAL COOPERATIVES (BAAC) | BAACBank |
THANACHART BANK | TTBBank |
GOVERNMENT SAVINGS BANK | GSBBank |
CIMB THAI | CimbBankTH |
LAND AND HOUSES BANK | LHBank |
KIATNAKIN PHATRA BANK | KKPBank |
GOVERNMENT HOUSING BANK | GHBank |
UOB THAI | UOBBankTH |
ISLAMIC BANK OF THAILAND | IBank |
STANDARD CHARTERED BANK (THAI) | SCBankTH |
TISCO BANK | TiscoBank |
CITIBANK THAI | CitiBankTH |
HSBC BANK (THAILAND) | HSBCBankTH |
THAI CREDIT RETAIL BANK | TCRBank |
ICBC THAI | ICBCBankTH |
SME DEVELOPMENT BANK OF THAILAND | SMEDevBank |
EXPORT–IMPORT BANK OF THAILAND | ExImBank |
QRPAY
Bank | Code |
---|---|
PROMPTPAY | PromptPay |
SCB PROMPTPAY QR | PromptPaySCB |
E-WALLET
Bank | Code |
---|---|
TRUEMONEY | TrueMoney |
Indonesia
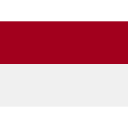
BANK TRANSFER
Bank | Code |
---|---|
MANDIRI BANK | MandiriBank |
CIMB NIAGA | CimbNiagaBank |
BANK CENTRAL ASIA (BCA) | BCA |
BANK RAKYAT INDONESIA (BRI) | BRI |
BANK NEGARA INDONESIA (BNI) | BNI |
BANK DANAMON | DanamonBank |
PERMATA BANK | PermataBank |
BANK MEGA | BMI |
BANK SINARMAS | BSM |
BANK TABUNGAN NEGARA | BTN |
OCBC NISP | OCBCBankID |
PT BANK PAN INDONESIA | PaninBank |
UOB BANK (INDONESIA) | UOBBankID |
BANK SYARIAH INDONESIA | BSI |
PT BANK COMMONWEALTH | CommBank |
HSBC BANK (INDONESIA) | HSBCBankID |
DBS BANK (INDONESIA) | DBSBankID |
HANA BANK (INDONESIA) | HanaBankID |
BANK MNC INTERNASIONAL | MNCBank |
BANK KALTENG | BankKalteng |
ALLO BANK INDONESIA | AlloBank |
SEABANK (INDONESIA) | SeaBankID |
BANK TABUNGAN PENSIUNAN NASIONAL | BTPN |
BANK MAYBANK INDONESIA | MayBankID |
QRPAY
Bank | Code |
---|---|
QRIS | Qris |
DANA | DANA |
OVO | OVO |
LINKAJA | LinkAja |
Philippines
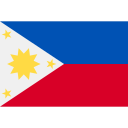
QRPAY
Bank | Code |
---|---|
QR PH | QRPh |
GCASH | Gcash |
BANK TRANSFER
Bank | Code |
---|---|
BANK OF THE PHILIPPINE ISLANDS (BPI) | BPI |
UNIONBANK OF THE PHILIPPINES | UnionBankPH |
RIZAL COMMERCIAL BANKING CORPORATION (RCBC) | RCBCBank |
METROPOLITAN BANK & TRUST COMPANY (METROBANK) | MetroBank |
SEABANK PHILIPPINES | SeaBankPH |
Singapore
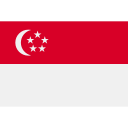
BANK TRANSFER
Bank | Code |
---|---|
DBS BANK | DBSBank |
STANDARD CHARTERED BANK (SINGAPORE) | SCBankSG |
UOB BANK (SINGAPORE) | UOBBankSG |
OCBC BANK | OCBCBankSG |
PAYNOW | Paynow |
HSBC BANK (SINGAPORE) | HSBCBankSG |
Bangladesh
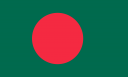
QRPAY
Bank | Code |
---|---|
NAGAD | Nagad |
BKASH OB | Bkash-OB |
Nepal
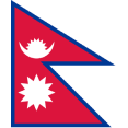
BANK TRANSFER
Bank | Code |
---|---|
MACHBANK | MachBank |
MANJUSHREE FINANCE LIMITED | MFLBank |
SINDHU BIKASH BANK | SBBank |
ADBL | ADBL |
SANIMA BANK | SanimaBank |
CITIZENS BANK INTERNATIONAL LIMITED | CBILBank |
PRIME COMMERCIAL BANK | PCBank |
LAXMI LAGHUBITTA BITTIYA SANSTHA LIMITED | LaxmiLBSLBank |
NIC ASIA BANK | NicAsiaBank |
NAVAJEEVAN COOPERATIVE LTD. | NCLBank |
MITERI DEVELOPMENT BANK LIMITED | MDBLBank |
GREEN DEVELOPMENT BANK LIMITED | GDBLBank |
SAPTAKOSHI DEVELOPMENT BANK | SDBank |
SAHARA NEPAL CO-OPERATIVE LTD. | SNCLBank |
ESEWA | ESEWA |
FONEPAY | Fonepay |
NMB BANK LIMITED | NMBBank |
PROGRESSIVE FINANCE LIMITED | PFLBank |
IME CO-OPERATIVE | ImeCoop |
KISAN SAVING AND CREDIT COOPERATIVE LIMITED | KSCCLBank |
POKHARA FINANCE LIMITED | PokharaBank |
KARNALI DEVELOPMENT BANK | KarnaliBank |
MUKTINATH BIKASH BANK | MBBank |
ESEWA REMIT | ESEWARemit |
SRIJANA FINANCE LIMITED | SFLBank |
CIVIL BANK LIMITED | CBLBank |
LAXMI BANK | LaxmiBank |
LUMBINI BIKASH BANK LIMITED | LBBLBank |
GUHESWORI MERCHANT BANKING & FINANCE | GMBFBank |
SHINE RESUNGA DEVELOPMENT BANK | SRDBank |
STAR SAVING AND CREDIT COOPERATIVE LIMITED | StarSCCLBank |
GOODWILL FINANCE LIMITED | GWFLBank |
EVEREST BANK LIMITED | EBLBank |
MULTIPURPOSE FINANCE LIMITED | MPFLBank |
RELIANCE FINANCE | RFBank |
SUNRISE BANK | SunriseBank |
NEPAL MULTIPURPOSE CO-OPERATIVE SOCIETY LTD. | NMCSLBank |
NEPAL CREDIT AND COMMERCE BANK LIMITED | NCCBLBank |
EXCEL DEVELOPMENT BANK | ExcelBank |
NAWAKANTIPUR | NawaBank |
MAHALAXMI BIKAS BANK | MLBBank |
KISAN BAHUUDESHIYA SAHAKARI SANSTHA LTD (MF) | KBSSLBank |
NEPAL BANK LIMITED | NBLBank |
NEPAL INVESTMENT MEGA BANK LTD. | NIMBank |
UNITED FINANCE LIMITED | UFLBank |
KAMANA SEWA BIKAS BANK | KSBBank |
GLOBAL IME BANK | GImeBank |
ICFC FINANCE | ICFCFinance |
RASTRIYA BANIJYA BANK | RBBank |
SAMRIDDHI FINANCE COMPANY LIMITED | SFCLBank |
SHREE INVESTMENT AND FINANCE COMPANY LTD | SIFCLBank |
ORANGENXT | OrangeNXT |
NEPAL FINANCE LIMITED | NFLBank |
CENTRAL FINANCE LIMITED | CFLBank |
PRABHU BANK | PrabhuBank |
GURKHAS FINANCE LIMITED | GKFLBank |
GARIMA BIKASH BANK | GBBank |
NEPAL SBI BANK LIMITED | NSBLBank |
SHANGRILA DEVELOPMENT BANK | ShangDBank |
NEPAL BANGLADESH BANK | NPBDBank |
BANK OF KATHMANDU | KathmanduBank |
BEST FINANCE LIMITED | BFLBank |
KUMARI BANK | KumariBank |
SIDDHARTHA BANK | SiddhaBank |
ROYAL SAVINGS | RoyalSavings |
CENTURY BANK LIMITED | CenturyBank |
JYOTI BIKASH BANK LIMITED | JBBLBank |
NABIL BANK | NabilBank |
Payment Bank
This is the list of payment methods that customer can choose to make transfer.
Malaysia
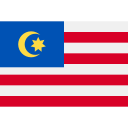
BANK TRANSFER
Bank | Code |
---|---|
PUBLIC BANK | PbeBank |
MAYBANK | MayBank |
CIMB BANK | CimbBank |
RHB BANK | RhbBank |
AM BANK | AmBank |
HONG LEONG BANK | HlbBank |
BANK SIMPANAN NASIONAL (BSN) | BSN |
ALLIANCE BANK | AllianceBank |
AFFIN BANK | AffinBank |
QRPAY
Bank | Code |
---|---|
TOUCH'N GO | Tng |
MAYBANK QRPAY | MayBankQR |
DUITNOW QR / E-WALLET PAYMENT | DuitNowQR |
RHB BANK QRPAY | RhbBankQR |
GRABPAY | GrabPay |
BOOST | Boost |
TELCO
Bank | Code |
---|---|
DIGI | Digi |
UMOBILE | Umobile |
MAXIS | Maxis |
CELCOM | Celcom |
TNG RELOAD PIN | TngRP |
Thailand
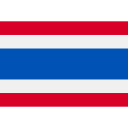
BANK TRANSFER
Bank | Code |
---|---|
KASIKORN BANK (KBANK) | KBank |
KRUNGSRI BANK (BAY) | KrungsriBank |
BANGKOK BANK | BangkokBank |
QRPAY
Bank | Code |
---|
E-WALLET
Bank | Code |
---|
Indonesia
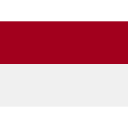
BANK TRANSFER
Bank | Code |
---|
QRPAY
Bank | Code |
---|---|
DANA | DANA |
OVO | OVO |
Philippines
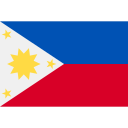
QRPAY
Bank | Code |
---|
BANK TRANSFER
Bank | Code |
---|
Singapore
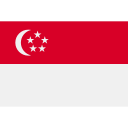
BANK TRANSFER
Bank | Code |
---|
Bangladesh
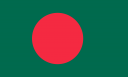
QRPAY
Bank | Code |
---|
Nepal
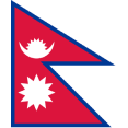
BANK TRANSFER
Bank | Code |
---|---|
MACHBANK | MachBank |
Withdraw Bank Table
This is the list of banks that available to withdraw.
Malaysia
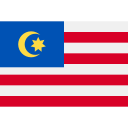
BANK TRANSFER
Bank | Code |
---|---|
MAYBANK | MayBank |
CIMB BANK | CimbBank |
RHB BANK | RhbBank |
BANK RAKYAT | BankRakyat |
BANK ISLAM | BankIslam |
AM BANK | AmBank |
HONG LEONG BANK | HlbBank |
BANK SIMPANAN NASIONAL (BSN) | BSN |
ALLIANCE BANK | AllianceBank |
AFFIN BANK | AffinBank |
HSBC BANK | HSBCBank |
STANDARD CHARTERED BANK | SCBank |
UOB BANK | UOBBank |
OCBC BANK | OCBCBank |
AL-RAJHI BANK | ARBank |
CITI BANK | CitiBank |
MBSB BANK | MBSBBank |
QRPAY
Bank | Code |
---|---|
TOUCH'N GO | Tng |
GXBANK | GXBank |
Thailand
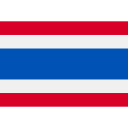
BANK TRANSFER
Bank | Code |
---|---|
KASIKORN BANK (KBANK) | KBank |
KRUNG THAI BANK (KTB) | KrungThaiBank |
SIAM COMMERCIAL BANK | SCBBank |
KRUNGSRI BANK (BAY) | KrungsriBank |
BANGKOK BANK | BangkokBank |
BANK FOR AGRICULTURE AND AGRICULTURAL COOPERATIVES (BAAC) | BAACBank |
THANACHART BANK | TTBBank |
GOVERNMENT SAVINGS BANK | GSBBank |
CIMB THAI | CimbBankTH |
LAND AND HOUSES BANK | LHBank |
KIATNAKIN PHATRA BANK | KKPBank |
GOVERNMENT HOUSING BANK | GHBank |
UOB THAI | UOBBankTH |
ISLAMIC BANK OF THAILAND | IBank |
STANDARD CHARTERED BANK (THAI) | SCBankTH |
TISCO BANK | TiscoBank |
CITIBANK THAI | CitiBankTH |
HSBC BANK (THAILAND) | HSBCBankTH |
THAI CREDIT RETAIL BANK | TCRBank |
ICBC THAI | ICBCBankTH |
E-WALLET
Bank | Code |
---|---|
TRUEMONEY | TrueMoney |
Indonesia
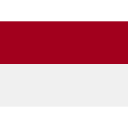
BANK TRANSFER
Bank | Code |
---|---|
MANDIRI BANK | MandiriBank |
CIMB NIAGA | CimbNiagaBank |
BANK CENTRAL ASIA (BCA) | BCA |
BANK RAKYAT INDONESIA (BRI) | BRI |
BANK NEGARA INDONESIA (BNI) | BNI |
BANK DANAMON | DanamonBank |
PERMATA BANK | PermataBank |
BANK MEGA | BMI |
BANK SINARMAS | BSM |
BANK TABUNGAN NEGARA | BTN |
OCBC NISP | OCBCBankID |
PT BANK PAN INDONESIA | PaninBank |
UOB BANK (INDONESIA) | UOBBankID |
BANK SYARIAH INDONESIA | BSI |
PT BANK COMMONWEALTH | CommBank |
HSBC BANK (INDONESIA) | HSBCBankID |
DBS BANK (INDONESIA) | DBSBankID |
HANA BANK (INDONESIA) | HanaBankID |
BANK MNC INTERNASIONAL | MNCBank |
BANK KALTENG | BankKalteng |
ALLO BANK INDONESIA | AlloBank |
SEABANK (INDONESIA) | SeaBankID |
BANK TABUNGAN PENSIUNAN NASIONAL | BTPN |
BANK MAYBANK INDONESIA | MayBankID |
Philippines
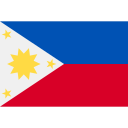
QRPAY
Bank | Code |
---|---|
GCASH | Gcash |